Visual Unity Debugging with ALINE
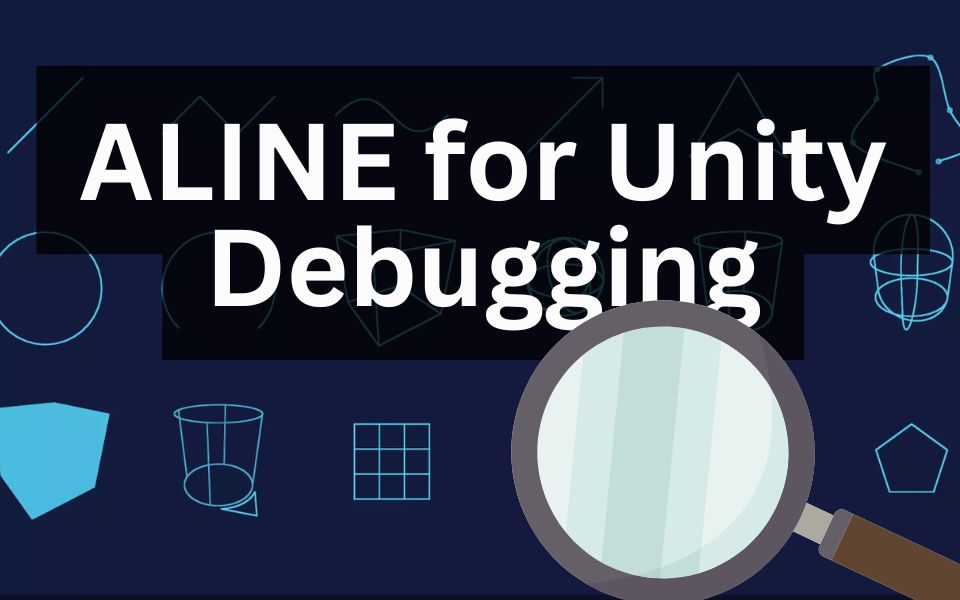
In Unity game development, debugging is often time-consuming, especially when it comes to visualizing game data and interactions in real-time. If you’ve ever found yourself trying to debug game logic such as unit pathing, combat, or other in-game mechanics, you know it can become a juggling act between coding and visualization. The debugger and log statements won’t help much here and Unity gizmos and Debug.Draw are a bit limited. This is where ALINE, a Unity asset by Aron Granberg for drawing and debugging can really simplify your workflow.
What is ALINE?
ALINE is a complete replacement asset for Unity’s built-in Debug.Draw and Gizmo drawing functionality and all your other drawing needs. It is faster, has more features and has better rendering quality. It was created by Aron Granberg (the creator of the popular A* Pathfinding Project) and provides an easy to use API for drawing and gizmos.
You can check out the full description of features on the asset store page but here’s an overview:
- More drawing primitives
- Simple, flexible and consistent API
- High performance. Often outperforming Unity’s APIs significantly
- Draw from inside Burst/ECS jobs, making debugging a breeze.
- Render in standalone games. Excellent if you want to make a level editor, or have a sci-fi look in your game.
Here’s some of the primitives the API supports:
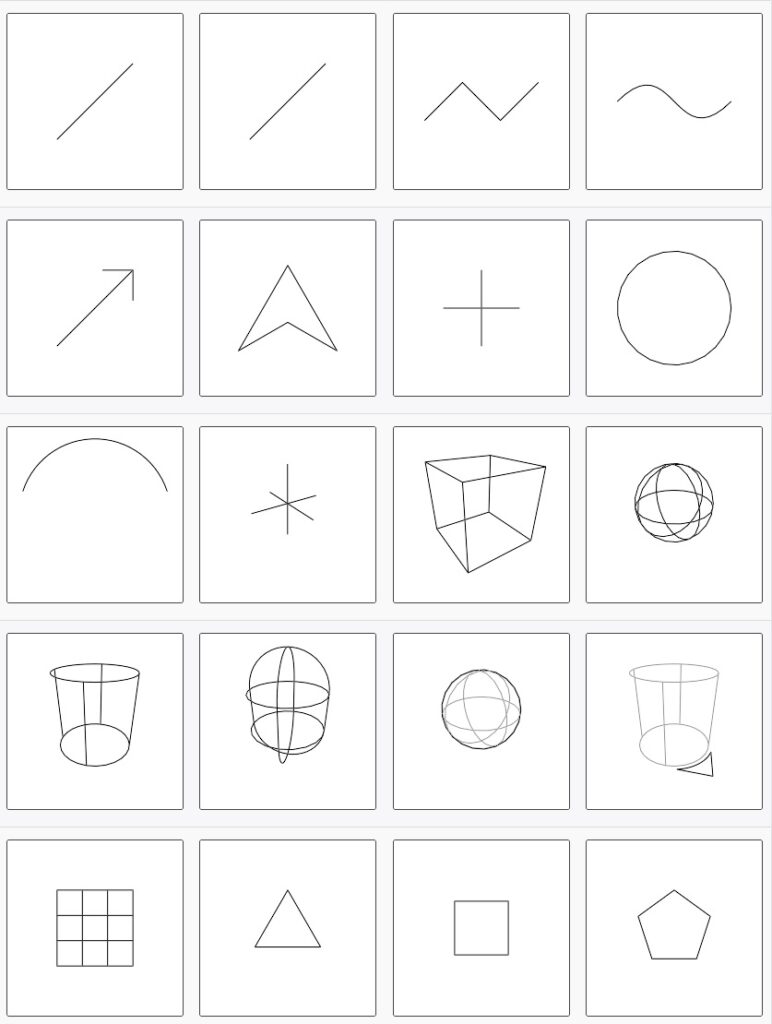
Using ALINE to Improve Your Dev Workflow
I primarily use ALINE to draw visuals for debugging (replacement for Gizmos or Debug.Draw). Things like spawn points, unit path finding and targeting, or visualizing line of sight.
It’s incredibly easy to do this with just 1 line of code. Here’s an example of drawing from Update()
void Update () {
// Draw a cylinder at the object's position with a height of 2 and a radius of 0.5
Draw.WireCylinder(transform.position, Vector3.up, 2f, 0.5f);
}
There’s also a convenient replacement for Unity’s OnGizmoDraw
public override void DrawGizmos () {
using (Draw.InLocalSpace(transform)) {
// Draw a cylinder at the object's position with a height of 2 and a radius of 0.5
Draw.WireCylinder(Vector3.zero, Vector3.up, 2f, 0.5f);
}
}
In my project I have enemy spawners that are defined via parameters such as rows, columns, spacing, etc and I wanted to be able to visualize them in the scene.
I used the following to draw the individual unit positions:
public override void DrawGizmos()
{
var countPerRow = _count / _rows;
var centerOffset = new Vector3(countPerRow / 2f * _spacing, 0, _rows / 2f * _spacing);
using (Draw.InLocalSpace(transform))
{
using (Draw.WithColor(IndexColors[Index]))
{
using (Draw.WithLineWidth(2))
{
for (var i = 0; i < _count; i++)
{
var row = i / countPerRow;
var column = i % countPerRow;
var localPosition = new Vector3(column * _spacing, 0.5f, row * _spacing) - centerOffset + _cubeOffset;
Draw.WireCylinder(localPosition, Vector3.up, 1, 0.5f);
}
}
}
}
}
Similar things can be done with Unity’s draw library but it does not look as good and doesn’t have as much customization (such as line width, easily draw in local space, etc)
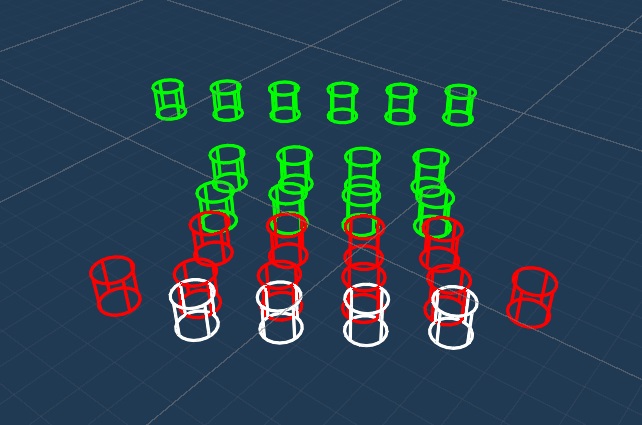
Final Thoughts
ALINE is a versatile tool for Unity developers, offering a significant improvement over Unity’s built-in Gizmos. Whether you’re working with AI, combat mechanics, or level design, ALINE’s enhanced drawing capabilities can save you countless hours, reduce frustration, and streamline your workflow.
If you’re looking to level up your debugging and improve productivity, give ALINE a try—it may just become an essential part of your Unity toolkit.
Interested in more assets to upgrade your Unity workflow? Check out my post on the best assets for boosting your Unity development productivity!
Jonathan
Hey there, I’m Jon! I love landscape photography which is why I travel all the time! On my blog I’ll share all the best spots for epic sunsets and sunrises as well as some photography tips and tricks. I also have a interest in game design so you’ll find the occasional post about that too. Thanks for visiting!